API documentation for libmpg123, libout123, and libsyn123
Note:
This API doc is automatically generated from the current development version that you can get via Subversion or as a daily snapshot from http://mpg123.org/snapshot.
There may be differences (additions) compared to the latest stable release. See
NEWS.libmpg123,
NEWS.libout123,
NEWS.libsyn123,
and the overall NEWS file on libmpg123 versions and important changes between them.
Let me emphasize that the policy for the lib*123 family is to always stay backwards compatible -- only additions are planned (and it's not yet planned to change the plans;-).
Let me emphasize that the policy for the lib*123 family is to always stay backwards compatible -- only additions are planned (and it's not yet planned to change the plans;-).
syn123.h File Reference
Include dependency graph for syn123.h:
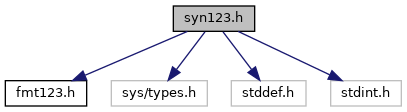
Go to the source code of this file.
Macros | |
#define | SYN123_API_VERSION 2 |
#define | SYN123_PATCHLEVEL 3 |
#define | MPG123_EXPORT |
#define | MPG123_RESTRICT |
#define | SYN123_DB_LIMIT 500 |
#define | SYN123_IOFF(sample, channel, channels) ((sample)*(channels)+(channel)) |
Typedefs | |
typedef struct syn123_struct | syn123_handle |
typedef ssize_t | syn123_ssize_t |
Enumerations | |
enum | syn123_error { SYN123_OK = 0 , SYN123_BAD_HANDLE , SYN123_BAD_FMT , SYN123_BAD_ENC , SYN123_BAD_CONV , SYN123_BAD_SIZE , SYN123_BAD_BUF , SYN123_BAD_CHOP , SYN123_DOOM , SYN123_WEIRD , SYN123_BAD_FREQ , SYN123_BAD_SWEEP , SYN123_OVERFLOW , SYN123_NO_DATA , SYN123_BAD_DATA } |
enum | syn123_wave_id { SYN123_WAVE_INVALID = -1 , SYN123_WAVE_FLAT = 0 , SYN123_WAVE_SINE , SYN123_WAVE_SQUARE , SYN123_WAVE_TRIANGLE , SYN123_WAVE_SAWTOOTH , SYN123_WAVE_GAUSS , SYN123_WAVE_PULSE , SYN123_WAVE_SHOT , SYN123_WAVE_LIMIT } |
enum | syn123_sweep_id { SYN123_SWEEP_LIN = 0 , SYN123_SWEEP_QUAD , SYN123_SWEEP_EXP , SYN123_SWEEP_LIMIT } |
Functions | |
MPG123_EXPORT const char * | syn123_distversion (unsigned int *major, unsigned int *minor, unsigned int *patch) |
MPG123_EXPORT unsigned int | syn123_libversion (unsigned int *patch) |
const char * | syn123_strerror (int errcode) |
MPG123_EXPORT syn123_handle * | syn123_new (long rate, int channels, int encoding, size_t maxbuf, int *err) |
MPG123_EXPORT void | syn123_del (syn123_handle *sh) |
MPG123_EXPORT int | syn123_dither (syn123_handle *sh, int dither, unsigned long *seed) |
MPG123_EXPORT size_t | syn123_read (syn123_handle *sh, void *dst, size_t dst_bytes) |
MPG123_EXPORT int | syn123_setup_waves (syn123_handle *sh, size_t count, int *id, double *freq, double *phase, int *backwards, size_t *period) |
MPG123_EXPORT int | syn123_query_waves (syn123_handle *sh, size_t *count, int *id, double *freq, double *phase, int *backwards, size_t *period) |
MPG123_EXPORT const char * | syn123_wave_name (int id) |
MPG123_EXPORT int | syn123_wave_id (const char *name) |
MPG123_EXPORT int | syn123_setup_sweep (syn123_handle *sh, int wave_id, double phase, int backwards, int sweep_id, double *f1, double *f2, int smooth, size_t duration, double *endphase, size_t *period, size_t *buffer_period) |
MPG123_EXPORT int | syn123_setup_pink (syn123_handle *sh, int rows, unsigned long seed, size_t *period) |
MPG123_EXPORT int | syn123_setup_white (syn123_handle *sh, unsigned long seed, size_t *period) |
MPG123_EXPORT int | syn123_setup_geiger (syn123_handle *sh, double activity, unsigned long seed, size_t *period) |
MPG123_EXPORT int | syn123_setup_silence (syn123_handle *sh) |
MPG123_EXPORT int | syn123_conv (void *MPG123_RESTRICT dst, int dst_enc, size_t dst_size, void *MPG123_RESTRICT src, int src_enc, size_t src_bytes, size_t *dst_bytes, size_t *clipped, syn123_handle *sh) |
MPG123_EXPORT double | syn123_db2lin (double db) |
MPG123_EXPORT double | syn123_lin2db (double volume) |
MPG123_EXPORT int | syn123_amp (void *buf, int encoding, size_t samples, double volume, double offset, size_t *clipped, syn123_handle *sh) |
MPG123_EXPORT size_t | syn123_clip (void *buf, int encoding, size_t samples) |
MPG123_EXPORT size_t | syn123_soft_clip (void *buf, int encoding, size_t samples, double limit, double width, syn123_handle *sh) |
MPG123_EXPORT void | syn123_interleave (void *MPG123_RESTRICT dst, void **MPG123_RESTRICT src, int channels, size_t samplesize, size_t samplecount) |
MPG123_EXPORT void | syn123_deinterleave (void **MPG123_RESTRICT dst, void *MPG123_RESTRICT src, int channels, size_t samplesize, size_t samplecount) |
MPG123_EXPORT void | syn123_mono2many (void *MPG123_RESTRICT dst, void *MPG123_RESTRICT src, int channels, size_t samplesize, size_t samplecount) |
MPG123_EXPORT int | syn123_mixenc (int src_enc, int dst_enc) |
MPG123_EXPORT int | syn123_mix (void *MPG123_RESTRICT dst, int dst_enc, int dst_channels, void *MPG123_RESTRICT src, int src_enc, int src_channels, const double *mixmatrix, size_t samples, int silence, size_t *clipped, syn123_handle *sh) |
MPG123_EXPORT int | syn123_setup_filter (syn123_handle *sh, int append, unsigned int order, double *b, double *a, int mixenc, int channels, int init_firstval) |
MPG123_EXPORT int | syn123_query_filter (syn123_handle *sh, size_t position, size_t *count, unsigned int *order, double *b, double *a, int *mixenc, int *channels, int *init_firstval) |
MPG123_EXPORT void | syn123_drop_filter (syn123_handle *sh, size_t count) |
MPG123_EXPORT int | syn123_filter (syn123_handle *sh, void *buf, int encoding, size_t samples) |
MPG123_EXPORT int | syn123_setup_resample (syn123_handle *sh, long inrate, long outrate, int channels, int dirty, int smooth) |
MPG123_EXPORT long | syn123_resample_maxrate (void) |
MPG123_EXPORT size_t | syn123_resample_count (long inrate, long outrate, size_t ins) |
MPG123_EXPORT size_t | syn123_resample_history (long inrate, long outrate, int dirty) |
MPG123_EXPORT size_t | syn123_resample_incount (long input_rate, long output_rate, size_t outs) |
MPG123_EXPORT size_t | syn123_resample_fillcount (long input_rate, long output_rate, size_t outs) |
MPG123_EXPORT size_t | syn123_resample_maxincount (long input_rate, long output_rate) |
MPG123_EXPORT size_t | syn123_resample_out (syn123_handle *sh, size_t ins, int *err) |
MPG123_EXPORT size_t | syn123_resample_in (syn123_handle *sh, size_t outs, int *err) |
MPG123_EXPORT int64_t | syn123_resample_total64 (long inrate, long outrate, int64_t ins) |
MPG123_EXPORT int64_t | syn123_resample_intotal64 (long inrate, long outrate, int64_t outs) |
MPG123_EXPORT size_t | syn123_resample (syn123_handle *sh, float *MPG123_RESTRICT dst, float *MPG123_RESTRICT src, size_t samples) |
MPG123_EXPORT void | syn123_swap_bytes (void *buf, size_t samplesize, size_t samplecount) |
MPG123_EXPORT void | syn123_host2le (void *buf, size_t samplesize, size_t samplecount) |
MPG123_EXPORT void | syn123_host2be (void *buf, size_t samplesize, size_t samplecount) |
MPG123_EXPORT void | syn123_le2host (void *buf, size_t samplesize, size_t samplecount) |
MPG123_EXPORT void | syn123_be2host (void *buf, size_t samplesize, size_t samplecount) |
MPG123_EXPORT syn123_ssize_t | syn123_resample_expect (syn123_handle *sh, size_t ins) |
MPG123_EXPORT syn123_ssize_t | syn123_resample_inexpect (syn123_handle *sh, size_t outs) |
MPG123_EXPORT off_t | syn123_resample_total (long inrate, long outrate, off_t ins) |
MPG123_EXPORT off_t | syn123_resample_intotal (long inrate, long outrate, off_t outs) |
Detailed Description
The header file for the libsyn123 library.
Definition in file syn123.h.
Macro Definition Documentation
◆ SYN123_API_VERSION
#define SYN123_API_VERSION 2 |
◆ SYN123_PATCHLEVEL
#define SYN123_PATCHLEVEL 3 |
◆ MPG123_EXPORT
#define MPG123_EXPORT |
Defines needed for MS Visual Studio(tm) DLL builds. Every public function must be prefixed with MPG123_EXPORT. When building the DLL ensure to define BUILD_MPG123_DLL. This makes the function accessible for clients and includes it in the import library which is created together with the DLL. When consuming the DLL ensure to define LINK_MPG123_DLL which imports the functions from the DLL.